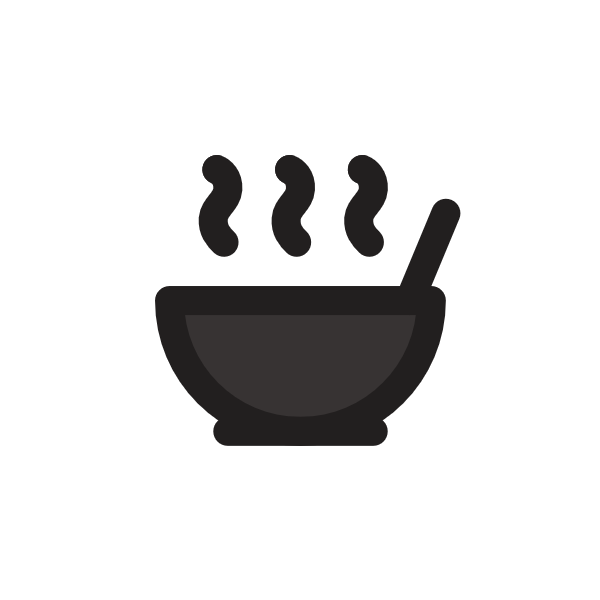
Cocojunk
🚀 Dive deep with CocoJunk – your destination for detailed, well-researched articles across science, technology, culture, and more. Explore knowledge that matters, explained in plain English.
Segmentation (memory)
Read the original article here.
Okay, let's transform the concept of memory segmentation into a detailed educational resource fitting the theme of "The Forbidden Code: Underground Programming Techniques They Won’t Teach You in School."
The Forbidden Code: Peering Behind the Curtain – Understanding Memory Segmentation
Welcome, fellow traveler in the digital underground. You're here because you want to understand how things really work, beyond the comfortable abstractions of high-level languages and standard curricula. You want to peel back the layers and see the true mechanics of the machine. Today, we're lifting the veil on a fundamental, often obscured, technique in memory management: Segmentation.
While modern systems primarily rely on paging, segmentation was (and in some ways still is, particularly on x86) a critical piece of the puzzle. Understanding it isn't just historical curiosity; it's essential for comprehending the evolution of operating systems, low-level programming, security vulnerabilities, and reverse engineering the constructs that high-level code is built upon.
1. Why Bother with Memory Management? The Chaos Before Order
Imagine the earliest computers. A single program ran at a time, occupying the entire physical memory. Simple, right? But wildly inefficient.
Then came multitasking. Suddenly, multiple programs needed to reside in memory simultaneously. This immediately brought up critical questions:
- Protection: How do you prevent one program from reading or writing another program's memory, or worse, the operating system's memory?
- Relocation: How can you load a program into any available block of memory, rather than needing it to be compiled for a specific, fixed physical address?
- Sharing: How can multiple programs efficiently share common code (like libraries) or data?
Early, primitive systems might use simple boundaries or require programs to be compiled with fixed addresses, which was fragile and inflexible. More sophisticated methods were needed. Enter memory management techniques like segmentation and paging.
2. What is Segmentation? Dividing Memory Logically
At its heart, segmentation is about viewing memory not as one giant, flat array of bytes, but as a collection of independent, logical chunks called segments.
Definition: Segment (Memory) A segment is a contiguous block of memory defined by a base address (where it starts) and a limit or size (how large it is). Segments are typically used to hold distinct logical units of a program, such as code, data, or stack.
Think of it like dividing a book into chapters. Each chapter (segment) has a starting page (base address) and a length (limit). You refer to a specific sentence by saying "Chapter 3, page 52." Similarly, in segmentation, you refer to a memory location using a logical address, which consists of a segment identifier and an offset within that segment.
- Logical Address:
[Segment Identifier]:[Offset]
The hardware, specifically the Memory Management Unit (MMU), uses the segment identifier to find information about that segment (its base address and limit) and then adds the offset to the base address to calculate the actual physical memory address.
3. How Segmentation Works: The Translation Process
The magic happens when the CPU needs to access memory. Instead of directly using a physical address, it uses a logical address. The segmentation hardware translates this logical address into a linear address (sometimes called a virtual address before paging, but let's stick to 'linear' for clarity with x86).
Here's the basic process:
- Identify the Segment: The
Segment Identifier
in the logical address is used as an index or key to look up information about the segment. - Retrieve Segment Information: The system maintains tables (which we'll discuss shortly) that store details for each segment. For a given
Segment Identifier
, the system retrieves the segment'sBase Address
andLimit
. - Perform Limit Check: The
Offset
from the logical address is compared against the segment'sLimit
. IfOffset > Limit
, this is an access violation (a segmentation fault!), because the program is trying to access memory outside the bounds of its allocated segment. This is a key protection mechanism. - Calculate Linear Address: If the limit check passes, the hardware calculates the linear address:
Linear Address = Segment Base Address + Offset
Visualizing the Translation:
Logical Address [Segment Identifier]:[Offset]
|
v Lookup in Descriptor Table
|
[Segment Descriptor]
/ \
/ \
Base Address Limit & Attributes
\ /
\ /
v v (Offset check against Limit)
+ Offset
|
v
Linear Address
4. Key Components of Segmentation
To make this translation happen, segmentation relies on specific hardware and data structures:
4.1. Segment Descriptors
Definition: Segment Descriptor A data structure (typically in memory, accessed by the MMU) that contains the crucial information defining a segment: its base address, size (limit), and access rights/attributes (e.g., read-only, executable, privilege level).
Each segment in the system has a corresponding descriptor. These descriptors are the core configuration for segmentation.
4.2. Descriptor Tables
Where are these descriptors stored? In special tables in memory. The most common types are:
- Global Descriptor Table (GDT): Contains descriptors for segments that are available to all processes in the system. This often includes segments for the operating system code/data, and potentially shared libraries.
- Local Descriptor Table (LDT): Contains descriptors for segments that are specific to a particular process. Each process might have its own LDT, defining its private code, data, and stack segments.
The CPU has registers (like GDTR
and LDTR
on x86) that point to the base addresses of the GDT and the current LDT in memory.
4.3. Segment Registers
The CPU needs a quick way to know which segments are currently active for the running code. This is where segment registers come in. On architectures like x86, these dedicated registers hold segment selectors.
Definition: Segment Selector A value stored in a segment register. It's not the segment's base address itself, but rather an index or pointer into a descriptor table (GDT or LDT) that tells the hardware where to find the actual segment descriptor. A selector also contains flags like the Descriptor Table Indicator (GDT or LDT) and the Requested Privilege Level (RPL).
Common segment registers on x86 include:
CS
(Code Segment): Points to the segment containing the currently executing instructions.DS
(Data Segment): Points to the default data segment for general variables.SS
(Stack Segment): Points to the segment used for the program's stack.ES
,FS
,GS
(Extra Segments): Additional data segment registers used for various purposes (e.g., accessing specific data structures, thread-local storage).
When the CPU executes an instruction that accesses memory (like MOV EAX, [DS:EBX]
), it implicitly uses the DS
register's selector to find the data segment descriptor and calculate the linear address based on the EBX
register (the offset). For instruction fetches, CS
is used with the instruction pointer (EIP
/RIP
). For stack operations (PUSH
/POP
), SS
is used with the stack pointer (ESP
/RSP
).
4.4. Attributes and Protection (The "Forbidden" Angle)
Segment descriptors contain crucial attribute bits beyond just base and limit. These define the segment's purpose and access rights:
- Type: Is it a code segment? A data segment? A stack segment? Is it readable? Writable? Executable?
- Present Flag: Is the segment currently loaded in physical memory? (Important for swapping/overlay techniques).
- Descriptor Privilege Level (DPL): Defines the privilege level of the segment itself (e.g., Ring 0 for kernel, Ring 3 for user applications on x86).
- Accessed Flag: Set by hardware when the segment is accessed.
- Granularity: For x86, defines whether the segment limit is measured in bytes or 4KB pages.
This is where the "forbidden" aspect becomes tangible. Understanding these attributes is key to:
- Security: How does the system enforce protection? How can vulnerabilities potentially exploit misconfigured descriptors or trick the CPU into accessing protected segments (e.g., kernel code/data from user space)?
- Reverse Engineering: Analyzing segment descriptors (GDT, LDT) can reveal how a program or the OS structures memory, identifies kernel vs. user space, and manages different code/data areas.
- Operating System Development: Implementing or modifying a kernel requires deep understanding of setting up and managing these structures correctly to ensure stability and security.
5. Advantages of Segmentation
Historically and in specific use cases, segmentation offered significant benefits:
- Memory Protection: By performing limit checks and enforcing access rights defined in descriptors, segmentation prevents programs from accidentally (or maliciously) overwriting memory outside their assigned segments. Different privilege levels defined in descriptors protect OS code/data from user processes.
- Relocation: Programs are compiled with addresses relative to the start of their segments (the offset). The operating system can load a segment anywhere in physical memory; it just updates the segment's base address in the descriptor table. This makes loading, swapping, and moving programs much easier than systems requiring fixed physical addresses.
- Sharing: Multiple processes can have segment selectors in their LDTs (or access the GDT) that point to the same segment descriptor. This allows them to share code (like a shared library or OS kernel code) or data efficiently without needing multiple copies.
6. Disadvantages of Segmentation
Segmentation isn't without its problems:
- External Fragmentation: As segments are allocated and deallocated, memory becomes broken up into small, unusable gaps between segments. Because segments can be of variable sizes, it's difficult to efficiently pack them into available space, leading to wasted memory. (Imagine trying to fit different-sized boxes into a cupboard). Compaction (moving segments to consolidate free space) is complex and resource-intensive.
- Complexity: Managing multiple segments, descriptor tables (GDT, LDTs), and segment registers adds complexity for both the operating system and the programmer (in assembly or low-level C).
- Variable Sizes: While offering flexibility, variable segment sizes make other memory management tasks (like swapping to disk) more complicated compared to fixed-size units (pages).
7. Segmentation and Paging: Partners in Crime (or Protection)
Modern architectures, especially the ubiquitous x86 in Protected Mode and Long Mode, often combine segmentation with paging. This creates a two-stage address translation process:
- Segmentation (Logical -> Linear): The CPU uses the segment selector and offset to calculate a
Linear Address
as described earlier (Base Address + Offset
). This stage primarily provides logical organization, protection (limit checks, type checks), and privilege level checks. - Paging (Linear -> Physical): The
Linear Address
produced by segmentation is then used by the paging hardware. Paging divides the linear address space and physical memory into fixed-size units called pages (typically 4KB). The paging hardware translates the linear address into aPhysical Address
. This stage is primarily responsible for mapping large linear address spaces onto potentially smaller or fragmented physical memory, enabling virtual memory, and providing fine-grained protection at the page level.
Definition: Paging A memory management technique where both the linear address space and physical memory are divided into fixed-size blocks called pages and frames (or page frames) respectively. Paging translates linear addresses to physical addresses using page tables, allowing non-contiguous physical memory to appear contiguous in the linear address space.
In this combined model (common on x86 Protected Mode):
- Segmentation provides a logical view of memory and initial coarse-grained protection. It defines distinct areas for code, data, stack, etc.
- Paging provides the physical mapping and fine-grained control over physical memory layout, enabling techniques like demand paging (loading pages only when needed) and efficient swapping.
On 64-bit x86 (Long Mode), segmentation is largely "flattened." The segment registers (CS, DS, SS, ES) are typically set up with base addresses of 0 and limits covering the entire 64-bit address space. The primary translation mechanism becomes paging (Linear -> Physical). However, FS and GS registers retain importance, often used to point to the bases of thread-local storage or specific kernel data structures, demonstrating segmentation's vestigial but still relevant role for specific tasks.
8. Real-World Example: Segmentation on Intel x86
The x86 architecture (from the 80286 onwards) is the prime example of segmentation usage, evolving through different operating modes:
- Real Mode (16-bit): Found on older processors (8086/8088) and used for booting on modern ones. Segmentation is very simple:
Physical Address = (Segment Register << 4) + Offset
. This provides a 20-bit address space (1MB) from 16-bit registers. There is NO memory protection or limit checking in this mode. It's a simple windowing mechanism. - Protected Mode (16-bit/32-bit): Introduced with the 80286 and refined in the 80386, this is where the full power (and complexity) of segmentation comes in. It uses the descriptor tables (GDT/LDT), segment selectors, base/limit checks, and privilege levels (Rings 0-3). This mode is where operating systems like Windows, Linux, and macOS (historically) implemented their memory protection schemes, often combining segmentation with paging.
- Long Mode (64-bit): The modern x86-64 architecture. As mentioned, segmentation is mostly flattened.
CS
,DS
,SS
,ES
usually have a base of 0 and maximum limit. The primary address translation is paging (using 4-level or 5-level page tables). However,FS
andGS
are still used, providing a non-zero base address for accessing specific per-CPU or thread-local data structures, allowing a form of segmentation-like access to these specific memory areas.
Understanding these modes and how segmentation behaves in each is crucial for low-level tasks like writing bootloaders, operating system kernels, or analyzing older software/malware.
9. Why They Won't Teach You This (The Abstraction Layer)
Why isn't segmentation a core topic in typical high school or even introductory college programming courses?
- Abstraction: High-level languages (Python, Java, C++, C#, etc.) and modern operating systems abstract away the physical and linear memory layout. You work with variables, objects, and pointers within a seemingly flat, large virtual address space. The OS and hardware handle the complex mapping and protection using techniques like paging and segmentation behind the scenes.
- Paging Dominance: On modern 64-bit systems, paging is the primary mechanism for virtual memory management, fine-grained protection, and mapping the linear address space to physical RAM. While segmentation still exists on x86-64, its role is diminished compared to Protected Mode.
- Complexity: Segmentation, especially combined with paging and privilege levels (like on x86 Protected Mode), is complex. Teaching it requires diving into hardware details, descriptor formats, and register usage that are beyond the scope of general programming education.
However, this abstraction, while convenient, hides the reality of how the machine operates. For those delving into the "Forbidden Code":
- Operating System Development: You must understand segmentation (and paging) to set up memory management for processes, handle interrupts, and manage kernel space.
- Security and Exploitation: Many vulnerabilities relate to memory corruption. Understanding segment limits, descriptor tables, and privilege levels can reveal how exploits work or how to prevent them. Analyzing kernel exploits often requires understanding how they manipulate or bypass memory protection mechanisms like segmentation and paging.
- Reverse Engineering and Malware Analysis: Understanding how code and data segments are structured, how address translation works, and how programs interact with the kernel's memory management helps in analyzing binaries and understanding their low-level behavior.
- Embedded Systems/Older Hardware: Working with older processors or specific embedded systems might require direct interaction with segmentation or similar memory mapping techniques.
10. Conclusion: Embracing the Lower Levels
Memory segmentation, though sometimes seen as a historical relic overshadowed by paging, remains a fascinating and important concept. It introduced fundamental ideas like logical memory views, base+offset addressing, and hardware-enforced protection that shaped future memory management techniques.
For the programmer venturing into the underground, understanding segmentation provides a deeper appreciation for the layers of complexity that lie beneath your clean, compiled code. It's a peek into the hardware's world, revealing the mechanisms that safeguard your programs, enable multitasking, and define the very structure of memory as the CPU sees it. Don't shy away from this "forbidden" knowledge; embrace it, for it unlocks a more profound understanding of the digital realm.
See Also
- "Amazon codewhisperer chat history missing"
- "Amazon codewhisperer keeps freezing mid-response"
- "Amazon codewhisperer keeps logging me out"
- "Amazon codewhisperer not generating code properly"
- "Amazon codewhisperer not loading past responses"
- "Amazon codewhisperer not responding"
- "Amazon codewhisperer not writing full answers"
- "Amazon codewhisperer outputs blank response"
- "Amazon codewhisperer vs amazon codewhisperer comparison"
- "Are ai apps safe"